Terraformをまったく知らないままAWSの世界に飛び込む前に、簡単にTerraformで遊んでおこうと思います。
そこでまず簡単なWebサーバーを立ち上げてみようと思います。
AWSとTerraformのセットアップ
AWSの資格情報を用意
- AWSコンソールからIAMに移動し、概要にあるアクセスキーを作成からアクセスキーIDとシークレットアクセスキーを取得し、開発マシンに設定します。僕はWSL2を使ったのでaws configureコマンドで設定しました。
aws configure
onishi@MYLOCALPC:~$ aws configure
AWS Access Key ID [None]: **************
AWS Secret Access Key [None]: *********************************
Default region name [None]: ap-southeast-2
Default output format [None]:
Terraformをインストール
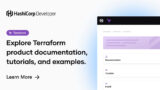
wget -O - https://apt.releases.hashicorp.com/gpg | sudo gpg --dearmor -o /usr/share/keyrings/hashicorp-archive-keyring.gpg
echo "deb [arch=$(dpkg --print-architecture) signed-by=/usr/share/keyrings/hashicorp-archive-keyring.gpg] https://apt.releases.hashicorp.com $(lsb_release -cs) main" | sudo tee /etc/apt/sources.list.d/hashicorp.list
sudo apt update && sudo apt install terraform
terraform公式サイトにあるインストール手順に沿ってインストールしました。
プロジェクトディレクトリの作成
適当なディレクトリを作り、そこでTerraformのファイルを管理します。
mkdir terraform-ec2-demo
cd terraform-ec2-demo
Terraformファイルの作成
最低限の構成として、**1つの.tf
ファイル(例: main.tf
)**にすべてまとめる形を示します。
※VPCやサブネットを自分で作りたい場合は、そこもTerraform管理にするのが理想ですが、最初はデフォルトVPCを使うとラクです。
############################################
# Terraform/AWS Provider設定
############################################
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
# v4.x系を使用(例)。なるべく新しいバージョンを指定してください。
version = "~> 4.0"
}
}
}
provider "aws" {
region = "ap-northeast-1" # 東京リージョンでの作成例
}
############################################
# デフォルトVPCの取得
############################################
data "aws_vpc" "default" {
default = true
}
############################################
# デフォルトサブネット一覧を取得 (aws_subnets)
############################################
data "aws_subnets" "default_subnets" {
# VPCを絞り込む
filter {
name = "vpc-id"
values = [data.aws_vpc.default.id]
}
# 「default-for-az = true」のタグが付いているサブネットだけを抽出
filter {
name = "default-for-az"
values = ["true"]
}
}
############################################
# セキュリティグループ (HTTPを許可)
############################################
resource "aws_security_group" "web_sg" {
name = "web-sg-demo"
description = "Allow HTTP inbound traffic"
vpc_id = data.aws_vpc.default.id
ingress {
description = "Allow HTTP from anywhere"
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
############################################
# EC2インスタンスの作成
############################################
resource "aws_instance" "web" {
ami = "ami-0c3fd0f5d33134a76" # Amazon Linux 2 (ap-northeast-1の一例)
instance_type = "t2.micro"
# デフォルトサブネットのうち、リストの先頭を使用(複数返る可能性があるため)
subnet_id = tolist(data.aws_subnets.default_subnets.ids)[0]
security_groups = [aws_security_group.web_sg.id]
# --- Webサーバの初期設定 ---
user_data = <<-EOF
#!/bin/bash
yum update -y
yum install -y httpd
systemctl start httpd
systemctl enable httpd
echo "<h1>Hello from Terraform on EC2!</h1>" > /var/www/html/index.html
EOF
tags = {
Name = "tf-demo-web"
}
}
############################################
# 出力
############################################
output "ec2_public_ip" {
value = aws_instance.web.public_ip
}
output "ec2_public_dns" {
value = aws_instance.web.public_dns
}
Terraformコマンドの実行
terraform init
まずは初期化コマンド。プロバイダのプラグインなどをダウンロードしてくれます。
terraform init
##出力
Terraform has been successfully initialized!
You may now begin working with Terraform. Try running "terraform plan" to see
any changes that are required for your infrastructure. All Terraform commands
should now work.
If you ever set or change modules or backend configuration for Terraform,
rerun this command to reinitialize your working directory. If you forget, other
commands will detect it and remind you to do so if necessary.
terraform plan
変更内容を確認するためのプランを表示します。
terraform plan
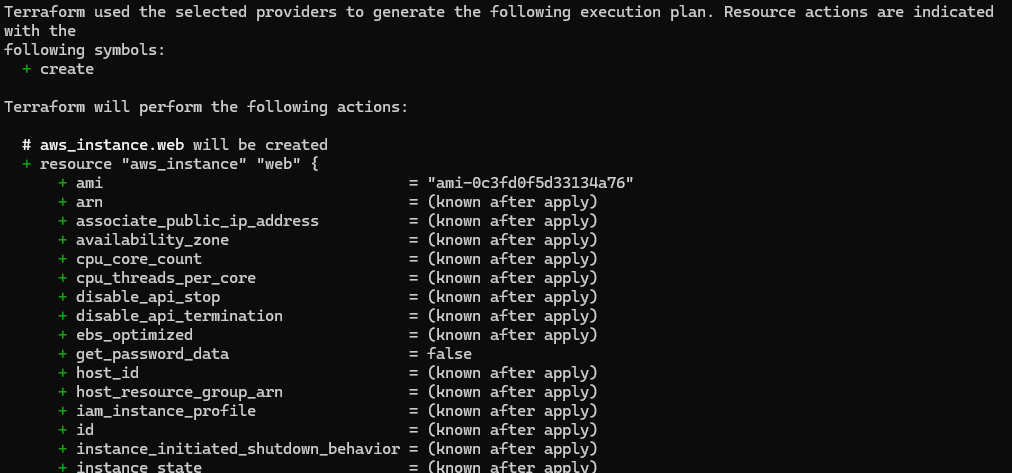
上記コマンドで「こんなリソースが作成されるよ」という差分が表示されます。エラーがなければOKです。
terraform apply
実際にAWS上にリソースを作成します。
terraform apply
#出力
Apply complete! Resources: 2 added, 0 changed, 0 destroyed.
Outputs:
ec2_public_dns = "ec2-**-***-***-**.ap-northeast-1.compute.amazonaws.com"
ec2_public_ip = "パブリックIPアドレス"
実行途中に「本当に適用してよいか?」と聞かれるので、yes
と入力すれば作成が始まります。
完了すると、出力に Apply complete! Resources: X added, 0 changed, 0 destroyed.
と表示され、先ほど output
で定義した public_ip
などが表示されるはずです。
動作確認
インスタンス作成まで少し時間がかかるので数分待ってから動作確認をします。
成功したのにインスタンスが見えない場合はリージョンを東京にしてみてください。
EC2に接続またはブラウザでチェック
- ブラウザを使用: 出力されたPublic IPまたはDNSに対して
http://<PUBLIC_IP>/
にアクセスします。- 例:
http://54.XXX.XXX.XXX/
- 成功すれば
Hello from Terraform on EC2!
のメッセージが表示されます。
- 例:
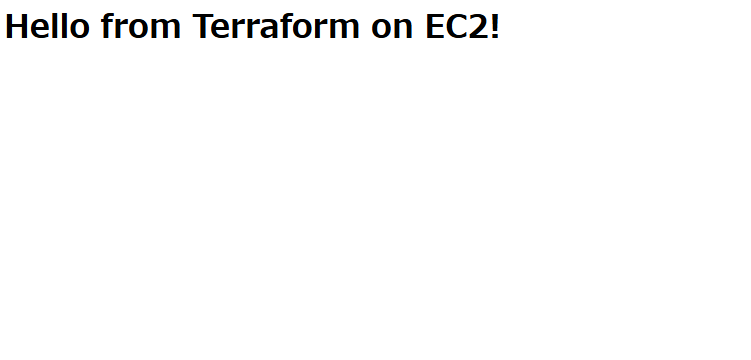
これで動作確認は完了です。
不要なので削除
お試しで実行しているインスタンスなので削除しておきます。
terraform destroy
terraformのいいところは作って終わりじゃなくて削除もできるところかもって思いました(他でもできるかもですが)
まとめ
今回は簡単にterraformについて学びましたが、もうちょっと開発環境を整えていろいろ見てみたいと思います。